What is the Difference between Server Side Rendering and Client Side Rendering
by Ayush Srivastava, System Analyst
Before we get into understanding what all these buzzwords mean, we should understand what "rendering" means. Rendering in web development means the process of turning application code into an interactive web page.
There are two types of rendering that we are going to discuss in this blog.
- Client Side Rendering
- Server Side Rendering
Client Side Rendering
Traditionally, all single-page web applications were rendered on the client-side. In a client side rendering process, the role of a server is limited to storing the client-side code of the application and data and transferring them to the browser when requested.
The user’s browser then compiles the code to turn it into an interactive application by adding functionality and generating the UI ( User Interface).
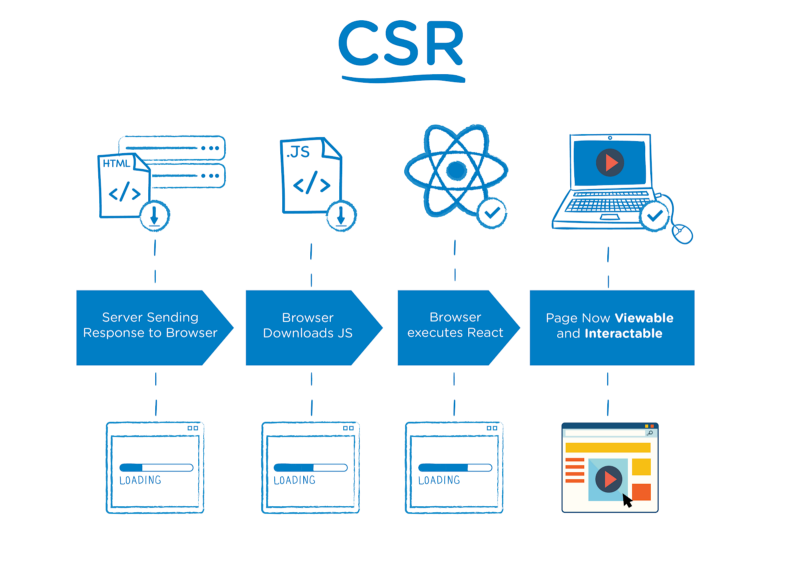
This is an HTML file sent to the browser in a client-side rendered application.
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>CSR</title>
</head>
<body>
<div id="root"><!-- blank --></div>
<script src="/bundle.js"></script>
</body>
</html>
Pros of Client Side rendering
Client side rendered sites are faster to navigate after the initial load since there are no more round trips to the server for every new page request.
Cons of Client Side rendering
- Initial time taken to display the content is large, which can be a bad user experience.
- CSR only sends the necessary data to the client, making it difficult for search engines to crawl and index the website's content.
Server Side Rendering
In Server Side rendering the static HTML markup is generated on the server so that the browser receives a rendered HTML page. The server receives the request from the client, retrieves the data, renders the page, and sends it back to the client. Now the browser does not need to render the page, so loading the static content is faster. The browser, however, still needs to load the javascript.
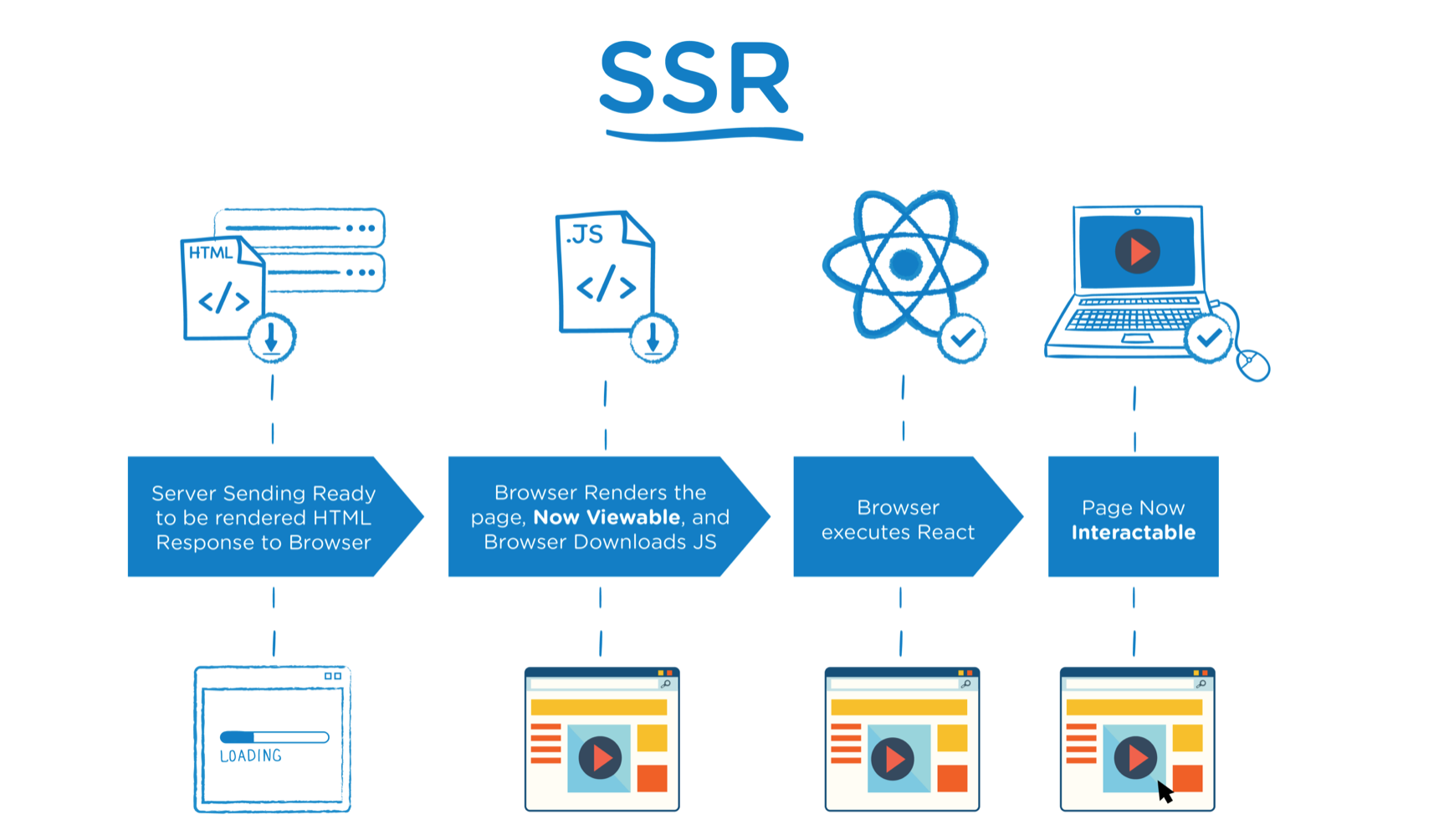
An example of HTML file, containing a simple static content, that browser received with server-side rendering. All HTML elements inside the root element were rendered on the server:
<!doctype html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<title>SSR</title>
</head>
<body>
<div id="root">
<div className="container">
<h2>Server Side Rendering</h2>
<p>This content was rendered on Server Side</p>
</div>
</div>
<script src="/bundle.js"></script>
</body>
</html>
Pros of Server Side rendering
- Better perceived performance: since the page is rendered on the server, transferred to the browser, and immediately visible, it feels quicker than seeing a blank page.
- Reduced latency: the database hosted on the server needs to serve the data to the server, which reduces the amount of time taken compared to if the data needed to travel to a browser located in some part of the world.
- SEO improves manifolds. Search engine bots can easily crawl static HTML sites.
Cons of Server Side Rendering
Server Side applications are expensive. For navigating to a new page every time, a new request has to be made to the server, which then fetches the data by making API calls and renders an HTML page for every request before passing it to the browser.
Popular Frameworks and Libraries offering CSR AND SSR
There are many popular front-end JavaScript frameworks that offer both Client Side Rendering (CSR) and Server Side Rendering (SSR) capabilities. Here are some examples:
-
React: React is a popular front-end JavaScript framework developed by Facebook that supports both CSR and SSR. React can be used to build single-page applications (SPAs) that use CSR, as well as server-rendered applications that use SSR.
-
Angular: Angular is a full-featured front-end JavaScript framework developed by Google that supports both CSR and SSR. Angular Universal is a package that allows developers to perform SSR with Angular.
-
Vue.js: Vue.js is a lightweight front-end JavaScript framework that supports both CSR and SSR. Vue.js can be used to build SPAs that use CSR, as well as server-rendered applications that use SSR.
-
Next.js: Next.js is a popular framework for building server-rendered React applications. It provides a simple and flexible API for rendering both static and dynamic content.
-
Nuxt.js: Nuxt.js is a framework for building server-rendered Vue.js applications. It provides a powerful configuration system that allows developers to customize the rendering process and optimize performance.
In summary, these frameworks provide the flexibility to choose between SSR and CSR, depending on the specific needs of the application. This makes it easier to build applications that are optimized for performance, SEO, and user experience.
Conclusion
Server Side Rendering (SSR) and Client Side Rendering (CSR) are two popular techniques for rendering web pages. SSR is better for websites that have a lot of dynamic content and need to be crawled by search engines. CSR is better for web applications that require fast and seamless user interactions. Ultimately, the choice between SSR and CSR depends on the website's specific needs and requirements.