Understanding Database Connections in Rails
by Giritharan, System Analyst
When developing a Rails application, configuring database credentials in the database.yml
file is just the first step in establishing a seamless connection between your application and the database. Let's dive into the technical details of what happens behind the scenes, from establishing a connection to managing multiple connections efficiently.
Configuring Database Credentials
The database.yml
file in a Rails application is where we specify the necessary credentials to connect to your database. These credentials typically include the adapter type (such as PostgreSQL), the database name, the username, the password, and the host.
development:
adapter: postgresql
encoding: unicode
database: myapp_development
pool: 5
username: myapp_user
password: password123
host: localhost
Establishing a TCP Connection: The Three-Way Handshake
Once the credentials are set, Rails, through ActiveRecord, begins the process of establishing a TCP connection to the database server. This involves the TCP three-way handshake:
-
SYN: The client sends a synchronization (SYN) packet to the server, requesting a connection.
-
SYN-ACK: The server responds with a synchronization-acknowledgment (SYN-ACK) packet, acknowledging the request.
-
ACK: The client sends an acknowledgment (ACK) packet back to the server, completing the handshake
Setting Session Parameters
After the TCP connection is established, the client and server exchange preferences and requirements to set the session parameters. This step ensures that both parties agree on certain aspects of the connection, such as character encoding and time zones.
Database Authentication
Next, the database performs authentication checks to verify the client's identity. PostgreSQL supports several authentication methods, including:
- Password (plaintext or MD5)
- GSSAPI
- SSPI
- And more
Once authenticated, the session is established, and the client can start sending commands to the server.
Sending Commands to the Server
With the connection established, Rails (via ActiveRecord) can now send SQL commands to the server. These commands are typically sent as simple text strings in the 'Query' message format. ActiveRecord translates Ruby method calls into SQL queries, which are then executed by the database.
Impact of Connection Numbers on Database Server
Managing the number of connections to the database is crucial for maintaining performance. Here's how the number of connections affects the server:
-
Increased CPU Usage: More connections lead to higher CPU usage as the server must manage more concurrent operations.
-
Memory Demand: Each connection consumes memory. More connections mean higher memory consumption, which can affect the server's overall performance.
-
Transaction Speed: Managing many connections can slow down transaction speeds, as the server's resources are spread thinner.
Exceeding Connection Limits in PostgreSQL
PostgreSQL has a configured limit on the number of connections it can handle. If the number of connection requests exceeds this limit, additional requests are rejected, preventing new clients from connecting to the database.
Connection Pooling in Rails
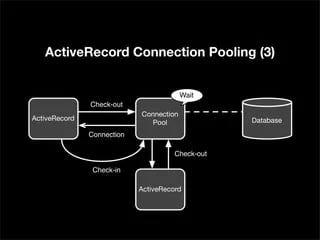
To manage database connections efficiently, Rails uses a technique called connection pooling. A connection pooler sits between the client (Rails application) and the database server, managing a pool of reusable connections. This approach has several benefits:
-
Resource Optimization: Connections are reused for multiple requests, reducing the overhead of establishing and closing connections frequently.
-
Improved Performance: By reusing connections, the pooler minimizes the time and resources needed to handle new requests.
-
Load Management: The pooler can handle multiple requests by distributing them across the available connections in the pool.
When a Rails application makes a database request, it connects to the connection pooler instead of directly to the database. The pooler interprets the queries, sends them to the database, receives the response, and then returns the response to the client. Once the response is returned, the connection is kept open in the pool for future use, rather than being closed immediately.
Understanding how databases connect to Rails applications is crucial for making them run well. It starts with setting up in database.yml
and involves steps like TCP handshakes, session parameters, authentication, and connection pooling. Each of these steps is important for smooth communication between your app and the database, especially when there's lots of activity. Proper management ensures your app stays fast and reliable.