Guide to set up Gitlab CI for Rails tests
by Menuba Tenny,
In this article, I'll walk you through the steps for setting up a rails application on Gitlab CI. We'll create a new application and configure it to execute tests on Gitlab CI.
Create a new ruby on rails application
$ rails new sample_rails_ci_setup
After creating the application you need to push it to Gitlab. Use the instructions on Gitlab to create new project and push the code.
Verify whether the test command is running successfully in the CLI by giving the command rails test
Create Configuration file for CI/CD pipeline
Gitlab uses a .gitlab-ci.yml
file for the instructions to be executed in the CI pipeline. Let us start by creating the file in the project's root directory.
Note: You can use YAML Linter to validate the .yml
file for proper indentation. (http://www.yamllint.com/)
Verify Successful execution using sample script
Let us check whether the Gitlab CI/CD pipeline is executing the commands in the .gitlab-ci.yml
by giving a sample script.
stages:
- build
- test
build-job:
stage: build
script:
- echo "Hello"
test-job:
stage: test
script:
- echo "This job tests something"
Here,
stages
are the steps that are involved in the execution. The commands under the build stage will be executed first and then the commands under the test stage will be executed.script
is where we write the commands that needs to be executed.
For better understanding, I have added two stages build
& test
. Since we are working on testing we will not use the build stage now.
Let's commit and push the changes to Gitlab and verify that both the build & test jobs execute successfully.
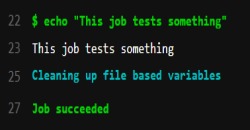
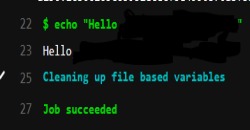
Sample script successfully executes in the pipeline.
Script for testing
Now that the sample code for test has been executed successfully, let's replace the script for testing the rails application by the command bundle exec rails test
.
stages:
- test
test-job:
stage: test
script:
- bundle exec rails test
Commit the changes and push it to Gitlab.
Next we get the following error
bundler: command not found: rails
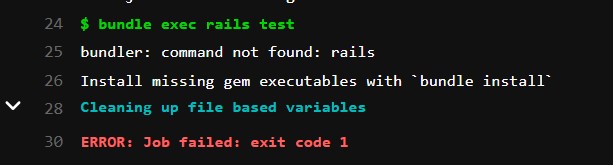
Install bundler
Let's install the bundler gem. Here I am specifying the version that matches my local bundler version. Also, in the next step give a command for bundle install
to install all gems from our Gemfile.
stages:
- test
test-job:
stage: test
script:
- gem install bundler -v 2.1.4
- bundle install
- bundle exec rails test
While running it shows:
Your Ruby version is 2.5.9, but your Gemfile specified 2.7.2

Use the correct ruby version
Seems like Gitlab currently uses ruby v2.5.9 by default. Since we are using ruby v2.7.2, let's specify CI to use the same ruby version for testing using the image key image: ruby:2.7.2
Before specifying the version just confirm which version you are currently using.
Command to check the version of ruby is ruby -v
stages:
- test
test-job:
stage: test
image: ruby:2.7.2
script:
- gem install bundler -v 2.1.4
- bundle install
- bundle exec rails test
Now, let's commit these changes to Gitlab and check whether the rails test command is running.
The tests execute successfully!
Set Postgresql as database for testing
Since we are using postgres in production, let's use the same in testing environment too. And also PostgreSQL is the most popular choice for Ruby on Rails projects.
stages:
- test
test-job:
stage: test
image: ruby:2.7.2
services:
- postgres:12.0
variables:
POSTGRES_USER: depot_postgresql
POSTGRES_PASSWORD: depot_postgresql
DB_USERNAME: depot_postgresql
DB_PASSWORD: depot_postgresql
DB_HOST: postgres
RAILS_ENV: test
DISABLE_SPRING: 1
BUNDLE_PATH: vendor/bundle
script:
- gem install bundler -v 2.1.4
- bundle install
- bundle exec rails db:create db:schema:load --trace
- bundle exec rails test
In this block of code we have included postgres service version 12.0 and ENV variables for setting up postgres user and database for testing.
The command bundle exec rails db:create db:schema:load --trace
will create a database and it will also load the database from schema.rb file.
Update the database.yml to use postgres instead of sqlite database
In your database.yml file,
test:
adapter: postgresql
encoding: unicode
pool: <%= ENV['DB_POOL'] %>
username: <%= ENV['DB_USERNAME'] %>
password: <%= ENV['DB_PASSWORD'] %>
host: <%= ENV['DB_HOST'] %>
port: 5432
database: depot_test
Now, push the changes to Gitlab
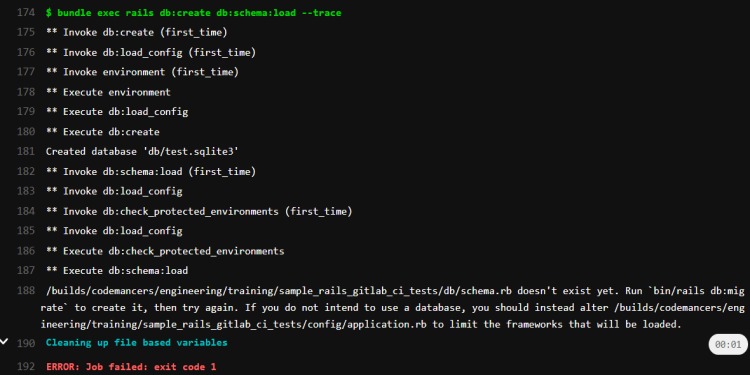
The above error occured because schema.rb file doesn't exist in db/config, let's create schema.rb by migrating the database.
Migrating the database
Now, we can give the command bundle exec rails db:migrate
to migrate the database.
stages:
- test
test-job:
stage: test
image: ruby:2.7.2
services:
- postgres:12.0
variables:
POSTGRES_USER: depot_postgresql
POSTGRES_PASSWORD: depot_postgresql
DB_USERNAME: depot_postgresql
DB_PASSWORD: depot_postgresql
DB_HOST: postgres
RAILS_ENV: test
DISABLE_SPRING: 1
BUNDLE_PATH: vendor/bundle
script:
- gem install bundler -v 2.1.4
- bundle install
- bundle exec rails db:migrate
- bundle exec rails db:create db:schema:load --trace
- bundle exec rails test
The tests execute successfully!

This was a basic TDD-like step by step setup guide to setting up rails tests on Gitlab CI.