Rails Inflections: The Magic Behind Singular and Plural Transformation
by Sujay Prabhu, Senior System Analyst
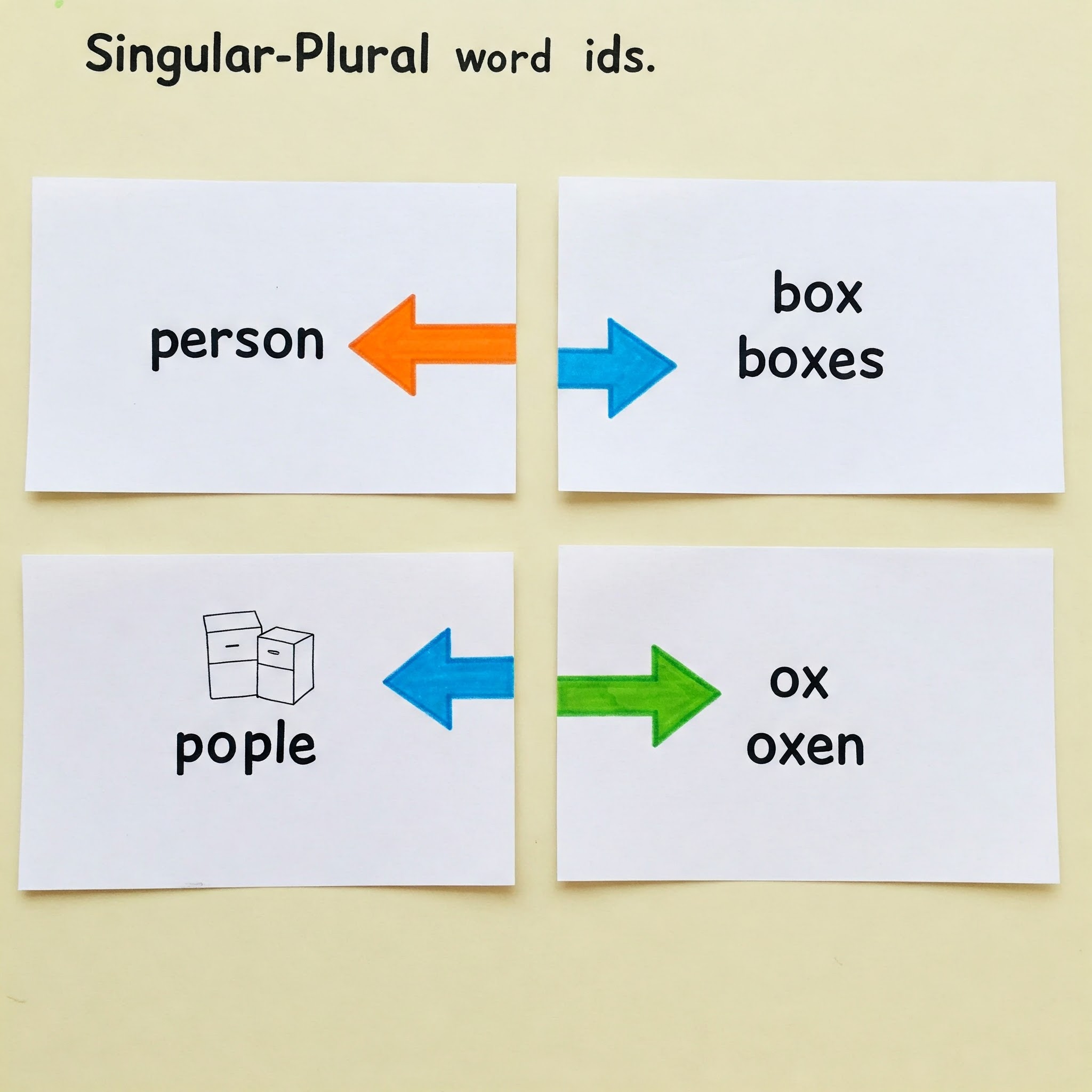
Let's start with a simple scaffold in Rails:
rails generate scaffold Person name:string email:string age:integer
This is what Rails generates:
invoke active_record
create db/migrate/20250427130336_create_people.rb
create app/models/person.rb
invoke test_unit
create test/models/person_test.rb
create test/fixtures/people.yml
invoke resource_route
route resources :people
invoke scaffold_controller
create app/controllers/people_controller.rb
invoke tailwindcss
create app/views/people
create app/views/people/index.html.erb
create app/views/people/edit.html.erb
create app/views/people/show.html.erb
create app/views/people/new.html.erb
create app/views/people/_form.html.erb
create app/views/people/_person.html.erb
invoke resource_route
invoke test_unit
create test/controllers/people_controller_test.rb
create test/system/people_test.rb
gsub test/system/people_test.rb
invoke helper
create app/helpers/people_helper.rb
invoke test_unit
invoke jbuilder
create app/views/people/index.json.jbuilder
create app/views/people/show.json.jbuilder
create app/views/people/_person.json.jbuilder
At first glance, everything seems fine, right? But take a closer look at the generated files. Notice how Rails automatically creates a people_controller
instead of a persons_controller
. How does it do this?
This is where things get interesting. English, as we all know, is filled with exceptions and irregularities. For example, the plural of “Person” is not “Persons” but “People”.
This might seem like a small detail, but Rails has built-in rules to handle these quirks automatically.
This isn't magic-it's Rails code in action. The tool responsible for handling such transformations is called Rails Inflector, which is part of the ActiveSupport library.
Inflector is smart enough to know how to pluralize and singularize words correctly, accounting for English language rules (and exceptions).
What is Rails Inflector?
Rails Inflector is a powerful tool that helps Rails understand how to pluralize and singularize words correctly. It’s like a dictionary of rules that Rails follows to make sure your code works properly when converting words between singular and plural forms.
Rails comes with a predefined set of rules that handle common English irregularities. These rules are defined in a file, which you can check out here.
Inflector exposes methods that you may have used, often without realizing their origin. Some common methods include:
-
humanize: Converts a string like "post_type" into "Post type."
-
pluralize: Turns a singular word into its plural form.
-
singularize: Turns a plural word into its singular form.
-
titleize: Capitalizes each word in a string.
-
capitalize: Capitalizes the first letter of a string.
If you want to dive deeper and explore all the available methods, you can check out the full source code in the Rails Github Repository
Customizing Inflections in Rails
While Rails already includes a set of inflection rules, you may come across certain words that don’t follow standard English pluralization rules.
Fortunately, Rails makes it easy to define custom rules in the config/initializers/inflections.rb
initializer.
Let’s take a look at a few ways you can customize Rails Inflector:
- Irregular
In English, some words have irregular plural forms, like "man" and "men," or "child" and "children". Rails Inflector lets you define such irregular pairs so that it knows how to handle them.
For example:
ActiveSupport::Inflector.inflections(:en) do |inflect|
inflect.irregular("appendix", "appendices")
end
Let’s test our new inflection in the Rails console by running rails c
(Rails console):
# Before
"appendix".pluralize # => "appendixes"
# After
"appendix".pluralize # => "appendices"
- Uncountable
Some words don’t have a plural form because they refer to something that can’t be counted individually, like "equipment" or "advice". Rails lets you specify these uncountable words so it doesn't try to pluralize them.
For example:
ActiveSupport::Inflector.inflections(:en) do |inflect|
inflect.uncountable("equipment")
end
Let’s test our new inflection in the Rails console
# Before
"equipment".pluralize # => "equipments"
# After
"equipment".pluralize # => "equipment"
- Acronyms
In the case of acronyms, Rails inflector ensures that your acronyms stay in their uppercase form. Here’s an example with 'HTML5' that demonstrates this behavior.
ActiveSupport::Inflector.inflections(:en) do |inflect|
inflect.acronym("HTML5")
end
# Before
"html5".camelize # => "Html5"
# After
"html5".camelize # => "HTML5"
In summary, Rails Inflections are a behind-the-scenes feature that handles a lot of the tricky grammar rules that come with converting between singular and plural forms.
Thanks to the power of ActiveSupport’s Inflector, Rails makes these transformations easy to implement and customize.
With just a few lines of code in the config/initializers/inflections.rb
initializer, you can handle everything from irregular plurals to uncountable nouns and acronyms.
So, next time you notice Rails automatically transforming your words, remember—it’s not magic, it’s just the power of Rails Inflections at work.